Use state in functions and create custom hooks.
We will learn how can we start using state in functional components and declare our own custom hooks to scale our react application
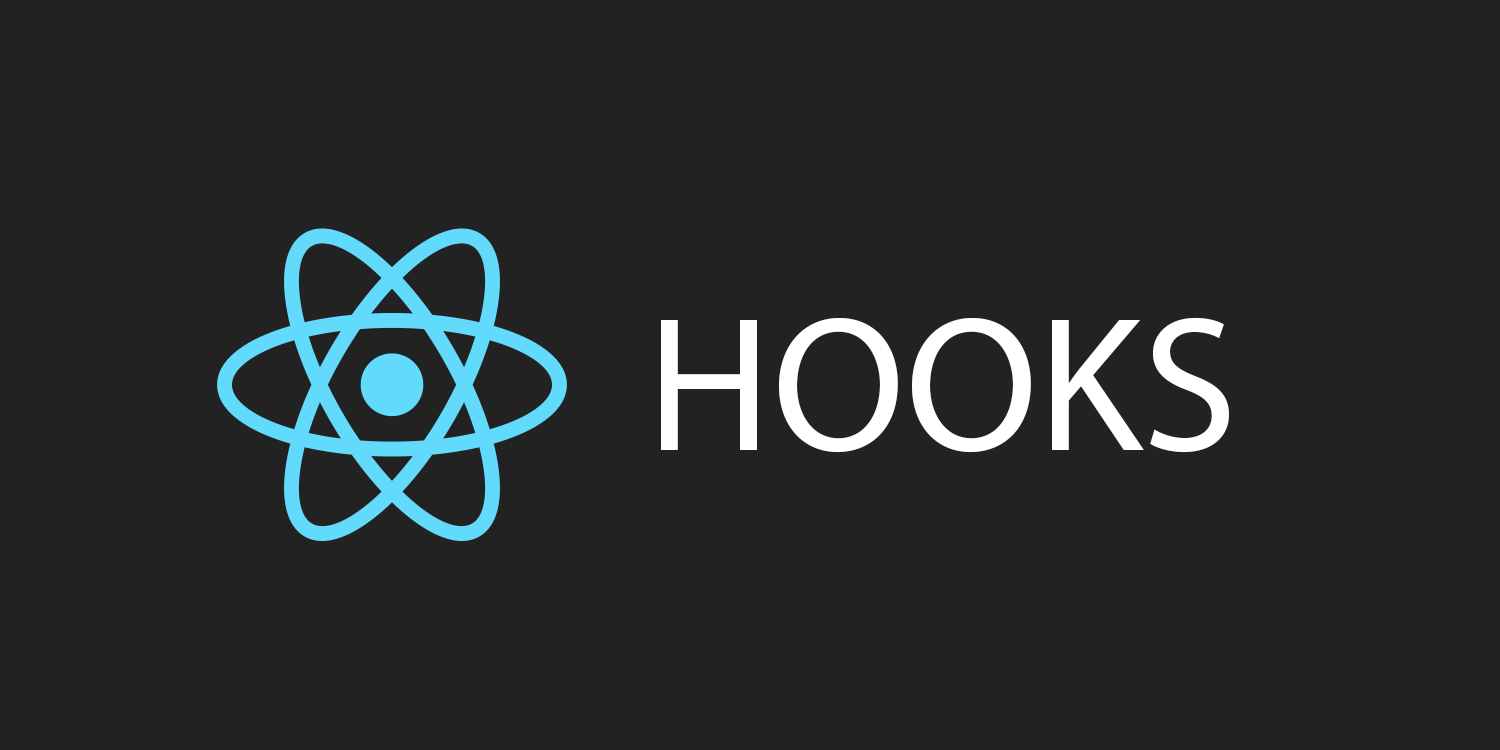
Let's create a functional component and use the useState hook from react.
useState will return an array with 2 values inside [state,setter]
Using the setter you can change the value of the state. Below is an example of a counter component with increment method that calls our setCount
which is our state setter and increments the value by 1.
function ExampleComponent = (props) => {
const [count, setCount] = useState(0);
inc = () => {
setCount(++count)
}
return (
<div>
<p>Current count: {count} </p>
<button onClick={inc}>Increment</button>
</div>
)
}
We can also create custom hook in order to keep our code smaller, maintainable and scaleable.
We define useCountHook
this function we receive initial state and will return an array similar to useState with 2 values [state, method to generate new state]
.
This allows us to separate the logic from our main component and let the custom hook handle the business logic.
function useCountHook = (initial) => {
const [count, setCount] = useState(initial);
inc = () => {
setCount(++count)
}
return [count,inc]
}
function ExampleComponent = (props) => {
const [count, increment] = useCountHook(0)
return (
<div>
<p>Current count: {count} </p>
<button onClick={increment}>Increment</button>
</div>
)
}
That's all.