TensorFlow in Node.js
Learn how to use TensorFlow.js, a machine learning library, in a Node.js application. We provide examples and a guide for building and training models, as well as tips for using TensorFlow.js in production.
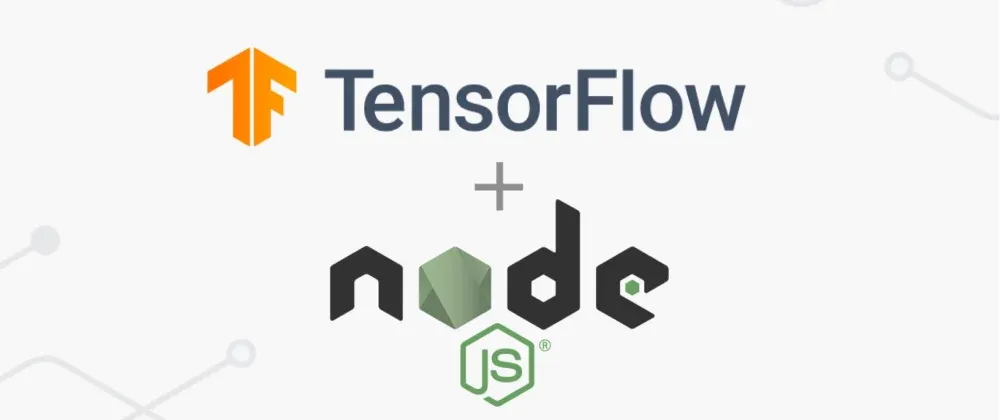
What is TensorFlow
TensorFlow is an open-source machine learning platform created by Google. It allows developers to create and train machine learning models, and deploy them in a variety of environments, including web applications. One such environment is Node.js, a popular JavaScript runtime that allows developers to build scalable network applications.
In this article, we will learn how to use TensorFlow in a Node.js application. We will start by explaining what TensorFlow is, and why it is useful for building machine learning models. We will then provide a simple example of how to use TensorFlow in Node.js, along with some documentation to help you get started.
One of the main benefits of TensorFlow is that it is highly flexible and can be used to build a wide variety of machine learning models, including deep learning models, convolutional neural networks, and natural language processing systems.
Why use TensorFlow in Node.js?
Node.js is a popular runtime environment for building web applications and other networked applications. It is particularly well-suited for building scalable, real-time applications, due to its non-blocking I/O model.
Using TensorFlow in a Node.js application can allow you to incorporate machine learning capabilities into your web application, giving you the ability to build intelligent, data-driven applications. This can be particularly useful for applications that need to analyze large amounts of data in real time, or for applications that need to make predictions based on input from users.
There are three main packages for using TensorFlow.js in Node.js:
@tensorflow/tfjs-node
: This package allows TensorFlow.js to be accelerated by the TensorFlow C binary and run on the CPU. It can be used on Linux, Windows, and Mac platforms. To install this package, runnpm install @tensorflow/tfjs-node
. You can then import the library into your Node.js application usingconst tf = require('@tensorflow/tfjs-node')
.@tensorflow/tfjs-node-gpu
: This package is similar to the CPU package, but it uses the GPU with CUDA to run tensor operations, resulting in faster performance. It is only available on Linux. To install this package, runnpm install @tensorflow/tfjs-node-gpu
. You can then import the library into your Node.js application usingconst tf = require('@tensorflow/tfjs-node-gpu')
.@tensorflow/tfjs
: This is the vanilla version of TensorFlow.js, which runs on the CPU using vanilla JavaScript. It does not rely on the TensorFlow binary and can be used on any platform that supports Node.js. To install this package, runnpm install @tensorflow/tfjs
. You can then import the library into your Node.js application usingconst tf = require('@tensorflow/tfjs')
.
Once you have imported the TensorFlow.js library, you can use it to build and train machine learning models just as you would in a web application. Here is a simple example of how to create a linear regression model using the CPU package:
const tf = require('@tensorflow/tfjs')
const xs = tf.tensor([1, 2, 3, 4]);
const ys = tf.tensor([1, 3, 5, 7]);
const model = tf.sequential();
model.add(tf.layers.dense({units: 1, inputShape: [1]}));
model.compile({optimizer: 'sgd', loss: 'meanSquaredError'});
model.fit(xs, ys, {epochs: 10}).then(() => {
model.predict(tf.tensor([5])).print();
});
This code creates a simple linear regression model that predicts a value based on a single input. The model is trained using the gradient descent optimization algorithm, with a mean squared error loss function.
The Node.js packages also provide additional APIs in the tf.node
namespace, such as tf.node.tensorBoard()
, which allows you to export summaries to TensorBoard in Node.js. Here is an example of how to use this API to export summaries of a model's training process:
const model = tf.sequential();
model.add(tf.layers.dense({ units: 1, inputShape: [200] }));
model.compile({
loss: 'meanSquaredError',
optimizer: 'sgd',
metrics: ['MAE']
});
// Generate some random fake data for demo purpose.
const xs = tf.randomUniform([10000, 200]);
const ys = tf.randomUniform([10000, 1]);
const valXs = tf.randomUniform([1000, 200]);
const valYs = tf.randomUniform([1000, 1]);
// Start model training process.
async function train() {
await model.fit(xs, ys, {
epochs: 100,
validationData: [valXs, valYs],
// Add the tensorBoard callback here.
callbacks: tf.node.tensorBoard('/tmp/fit_logs_1')
});
}
train();
This code trains a simple model with 100 epochs and exports summaries of the training process to TensorBoard.
It is important to note that the Node.js bindings for TensorFlow.js run synchronously, meaning that when you call an operation, it will block the main thread until the operation has been completed. This makes the bindings well-suited for scripts and offline tasks, but if you want to use TensorFlow.js in a production application, you should set up a job queue or worker threads to avoid blocking the main thread.
In summary, TensorFlow.js can be a powerful tool for building machine learning models in Node.js applications. By choosing the appropriate package and following best practices for production use, you can incorporate advanced machine-learning capabilities into your Node.js projects. With the proper documented examples and guides provided in this article, you should now have a good foundation for using TensorFlow.js in your Node.js projects.