Small import paths using aliases
How you can avoid using long import paths by using path aliases for shorter and more consistent imports in your projects.
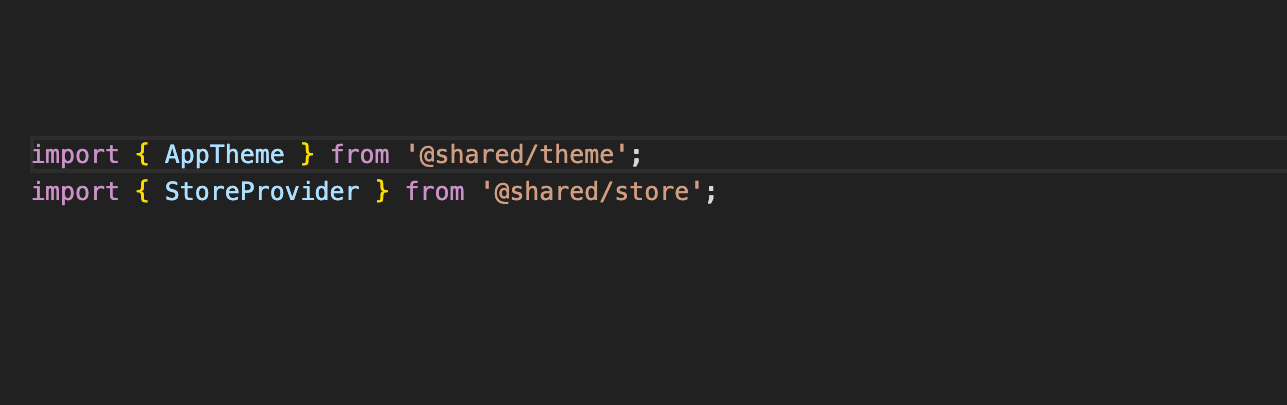
For someone like me, I truly hate to see long import paths and I hate to write them.
import { searchColumns } from '../../../../../core/components/ColumnPicker/ColumPicker.slice';
Many times we start importing from parent directories which make us write those ../
and even if we use the IDE feature of auto import we still going to see them.
Thankfully this is something we can solve easily with our project bundlers.
we can turn this '../../../../../core/components/ColumnPicker/ColumPicker.slice';
into this @components/ColumnPicker/ColumnPicker.slice
by adding something called path aliases.
Vite config
export default defineConfig({
plugins: [solidPlugin()],
resolve: {
alias: {
"@components": path.resolve(__dirname,'src/core/components'),
}
},
build: {
target: 'esnext',
polyfillDynamicImport: false,
},
});
Webpack config
const path = require('path');
module.exports = {
resolve: {
alias: {
"@components": path.resolve(__dirname,'src/core/components'),
}
}
// ... rest of your config
}
If you use typescript you must add your paths into tsconfig as well
{
"compilerOptions": {
...
"paths": {
"@components/*": ["./src/core/components/*"],
}
}
}
You can further shorten your import path using index.ts or .js
that handles exporting components in the current directory @components/ColumnPicker
export {searchColumns} from './ColumnPicker.slice'
Hope this small tweak improves your life as it improves mine.