Structuring a React Project for Scalability
Ensure your React project can grow and evolve with ease by following these principles of scalability: modular design, separation of concerns, clear naming conventions, and logical code organization. Get tips and best practices for structuring your project for long-term success.
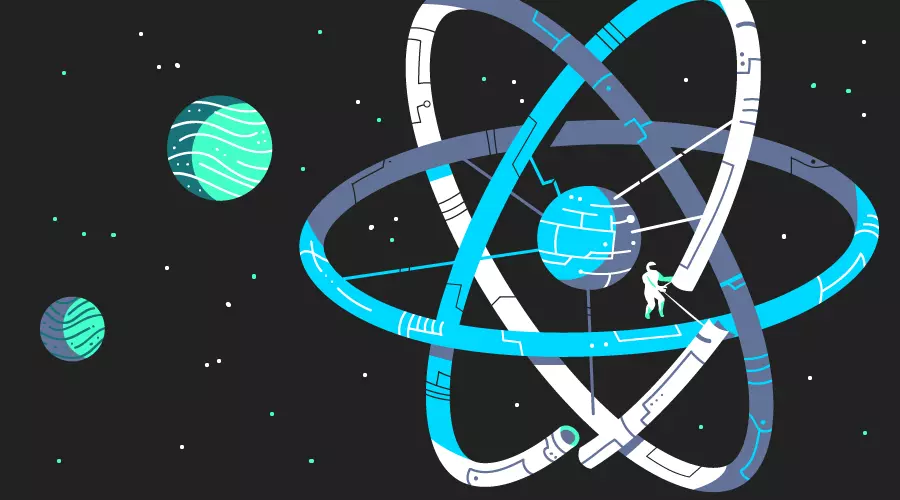
Structuring a React project for scalability is important to ensure that your application can grow and evolve over time without becoming unwieldy or difficult to maintain. There are a few key considerations to keep in mind when structuring a React project for scalability:
- Modular design: Dividing your application into small, self-contained modules makes it easier to reason about and maintain. It also makes it easier to reuse components and code across different parts of your application.
- Separation of concerns: Keeping related code together and separating unrelated code helps to make your application easier to understand and maintain. For example, you might want to keep your presentation components separate from your business logic.
- Naming conventions: Establishing clear and consistent naming conventions for your components and files can help to make your application more organized and easier to understand.
- Code organization: Organizing your code into a logical directory structure can help to make your application more maintainable. For example, you might want to create a separate directory for each major feature or component in your application.
- Test coverage: Ensuring that your application has good test coverage helps to ensure that the changes you make don't break existing functionality. This is especially important in a scalable application where changes can have a ripple effect throughout the codebase.
By following these principles, you can structure your React project in a way that is scalable, maintainable, and easy to understand. This will make it easier to add new features and make changes to your application as it grows and evolves.
How should your folders be structured to allow more team members to work on the project with fewer conflicts?
/src
/components
/common
/Button
Button.js
Button.css
/Header
Header.js
Header.css
/Footer
Footer.js
Footer.css
/feature1
/Feature1Component1
Feature1Component1.js
Feature1Component1.css
/Feature1Component2
Feature1Component2.js
Feature1Component2.css
/feature2
/Feature2Component1
Feature2Component1.js
Feature2Component1.css
/Feature2Component2
Feature2Component2.js
Feature2Component2.css
/images
/pages
/Home
Home.js
Home.css
/About
About.js
About.css
/Contact
Contact.js
Contact.css
/services
/feature1
api.js
/feature2
api.js
/store
/actions
actions.js
/reducers
reducers.js
/sagas
sagas.js
store.js
App.js
index.js
Here is a brief explanation of each of the folder types in the revised folder structure:
/components
: This directory contains the UI components that make up the application. It is further divided into subdirectories based on commonality or functionality (e.g./common
for components that are used throughout the application, or/feature1
for components specific to a particular feature)./images
: This directory contains any images that are used in the application or you can modify it to be generally static assets./pages
: This directory contains the top-level components for each page in the application./services
: This directory contains utility functions or API clients that are used by the application. It is further divided into subdirectories based on the feature or functionality that the services relate to./store
: This directory contains the code for the application's state management, including actions, reducers, sagas, and the store itself.App.js
: This file is the root component of the application and is responsible for rendering the other components.index.js
: This file is the entry point for the application and is responsible for rendering the root component to the DOM.
By organizing the code in this way, it is easy for team members to find the code they need to work on and make changes without affecting unrelated parts of the application. It also makes it easy to see the overall structure of the application and understand how different parts of the code fit together.
In conclusion, structuring a React project in a way that is scalable and maintainable is essential to ensure that your application can grow and evolve over time. By following principles such as modular design, separation of concerns, and clear naming conventions, you can create a project structure that is easy to understand and work with. This will make it easier to add new features and make changes to your application as it grows, ultimately saving you time and effort in the long run.
Limited time offer: Get Surfshark VPN at a discounted price
Looking for a reliable VPN to unblock content and enhance your online experience? Look no further! With impressive speeds and a secure connection, this is my personal top pick. Give it a try and see for yourself."