NestJs the future of Node web applications.
My thoughts on moving to Nestjs coming from express.
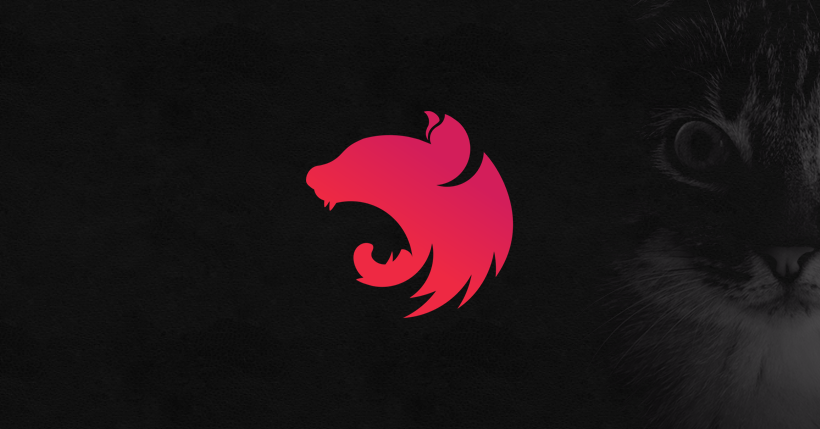
When building web applications using node.js, I would always start with express where I have deep knowledge and flexibility.
As years of experience go by I felt like it takes a lot of work to plan and structure ExpressJs for scale since it's an un-opinionated framework which means the framework trusts the developer to make the right choice and leaves lots of work to be done every time..
Recently I started to become more exposed to a framework called NestJS. I was very skeptical regarding this framework as it looks like a copy of angular.
I had the opportunity to test NestJs capabilities on a new home backend project.
Since I had prior experience with angular it was easy for me to adapt and by default, I write typescript so it wasn't match of hardship to quickly write an app with authentication and few endpoints.
I quickly discovered how NestJs is the perfect choice for developing highly maintainable and scalable applications.
Using typescript advanced features and futuristic javascript, You can write very scaleable and reusable code across your whole application.
@Controller('user')
export class UserController {
constructor(public userService: UserService) {}
@Get()
@Roles(Role.Admin)
allUsers() {
return this.userService.getAll();
}
@Get('profile')
getProfile(@Request() req) {
return req.user;
}
@Get(':username')
findByUsername(@Param('username') username: string) {
return this.userService.findOne(username);
}
}
In this example above, we have a controller which will handle all routes related to /api/user/
. We can see how decorators like @Get()
are used to defined the request method with ease or if we want to allow only specific role to access an endpoint, we can define our own custom decorator to validate user roles.
In the background, all these routes are accessible only with valid JWT
token which is configured globally by my own choice and if I decided I want to validate a single route and not globally, I could do that with ease using the same decorators pattern.
So what to do if all my endpoints are protected by default, how do we make an endpoint public?
If you though about another custom decorator, you are completely right. Since this is not tutorial for the framework but just showcase of some very useful features, here is another snippet
import { Controller, Post, Request, UseGuards } from '@nestjs/common';
import { AuthService } from './auth.service';
import { LocalAuthGuard } from './local-auth.guard';
import { Public } from './public.decorator';
@Controller('auth')
export class AuthController {
constructor(private authService: AuthService) {}
@UseGuards(LocalAuthGuard)
@Post('login')
@Public()
async login(@Request() req) {
return this.authService.login(req.user);
}
}
The controller above takes care of auth
route and has auth/login
route with post method. I won't explain the UseGuards
decorator you can read all about it with the NestJs extraordinary well written documentation.
We see that login
route is defined as @Public()
and therefore is accessible without JWT
token.
In summary, I am very impressed by how well the framework is written and how easy it is to move from express to NestJs while keeping good features like express middleware intact.
I highly recommend to check out this technology and I strongly believe that large scale applications written using node.js should be created using NestJs for ease of maintenance and scale.