How to use bootstrap in React
Our old fellow bootstrap can still be used in our React projects if wish so. In this short post we will learn how to use bootstrap in React application.
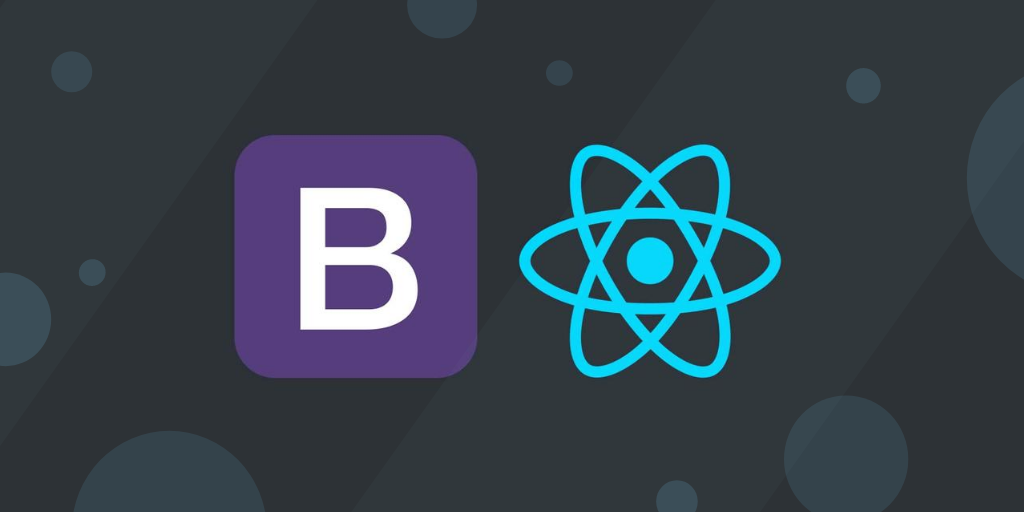
Let's start by creating a react project or using a project you already have.
You can follow the official docs on how to create a React project
Let's add bootstrap to our project
npm install react-bootstrap bootstrap
or
yarn add react-bootstrap bootstrap
To start using bootstrap in our React project we should go to the root of our project src/index.ts
to add the minimal CSS needed for bootstrap to look properly (if you use javascript then src/index.js
)
import React from 'react';
import ReactDOM from 'react-dom';
import 'bootstrap/dist/css/bootstrap.min.css';
import App from './App';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>
document.getElementById('root'),
);
Next, you can start importing bootstrap components to render into your react application
import Button from 'react-bootstrap/Button';
// or less ideally
import { Button } from 'react-bootstrap';
You should import individual components like:react-bootstrap/Button
rather than the entire library. Doing so pulls in only the specific components that you use, which can significantly reduce the amount of code you end up sending to the client.
https://react-bootstrap.github.io/getting-started/introduction
import React from 'react'
import Button from 'react-bootstrap/Button';
export default function App() {
<Button variant="primary">Primary Button</Button>
}
And you are done.