Exploring the Inner Workings of the Node.js Event Loop
Learn about the event loop, a central part of Node.js that manages async I/O and enables the runtime to handle a large number of concurrent connections with a small number of threads. With a deeper understanding of the event loop, you can build more efficient and scalable network applications.
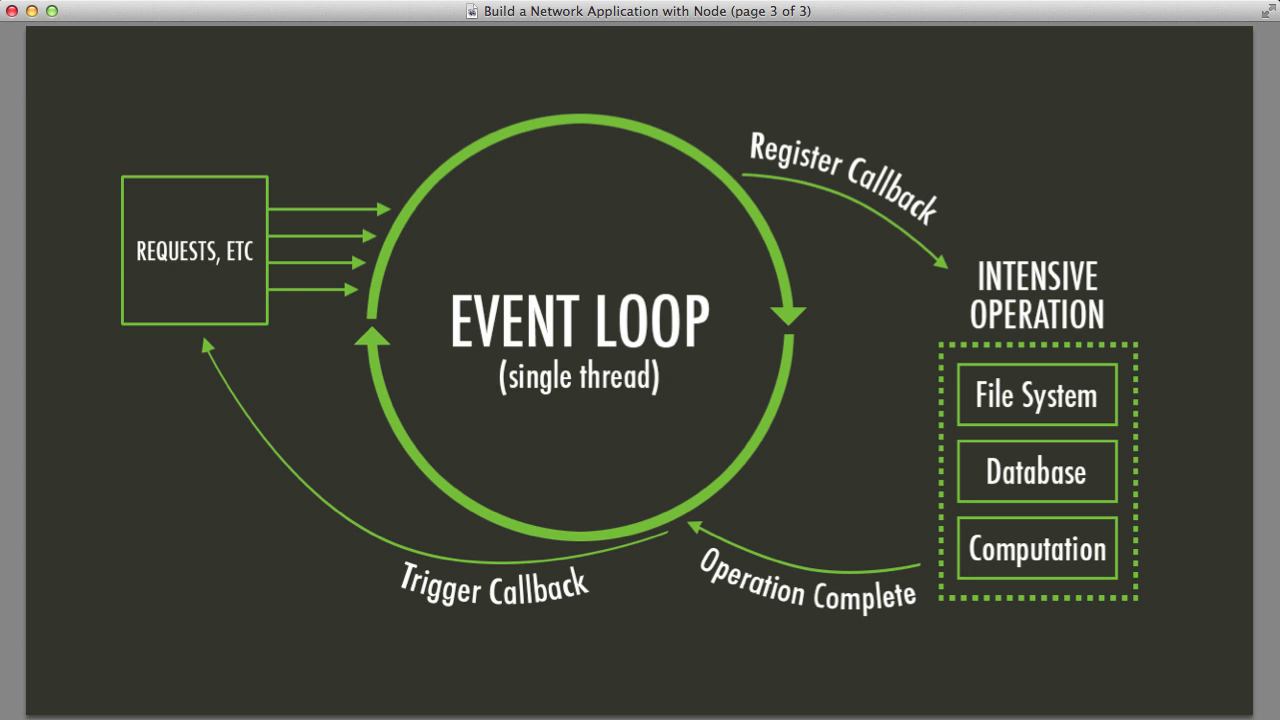
What is Node.js?
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine that allows developers to run JavaScript on the server side. One of the key features of Node.js is its event loop, which is responsible for managing asynchronous I/O operations in a non-blocking manner.
What is the event loop?
The event loop is a central part of Node.js and is what makes it possible for the runtime to handle a large number of concurrent connections with a small number of threads. It works by continuously executing a set of registered event handlers in a loop and dispatching new events to the appropriate handlers as they occur.
Here's a simple example of how the event loop works in Node.js:
const fs = require('fs');
fs.readFile('/file.txt', (err, data) => {
if (err) {
console.error(err);
} else {
console.log(data);
}
});
console.log('This code will run before the file is read.');
In this example, the readFile
function initiates an asynchronous read operation from the file system. When the operation completes, the callback function passed as an argument to readFile
is added to the event loop's queue of pending events. The event loop then continues to execute the rest of the code, including the console.log
statement.
Once all the synchronous code has been executed, the event loop enters its next iteration and checks the queue for pending events. If it finds any, it executes the associated event handlers and removes them from the queue. In this case, the callback function passed to readFile
will be executed, and the contents of /file.txt
will be logged to the console.
This simple example demonstrates how the event loop enables non-blocking I/O operations in Node.js. By allowing asynchronous operations to be registered as events and processed by the event loop, Node.js can efficiently handle a large number of concurrent requests without the overhead of creating and managing multiple threads.
For more information about the event loop in Node.js, you can check out the official Node.js documentation at https://nodejs.org/en/docs/guides/event-loop-timers-and-nexttick/. There are also many resources available online that provide more in-depth coverage of the event loop and its role in Node.js.