Revolutionizing Your Components with Custom React Hooks
In this blog post, you'll learn how to create your own custom React hooks and how they can help you share stateful logic across your application.
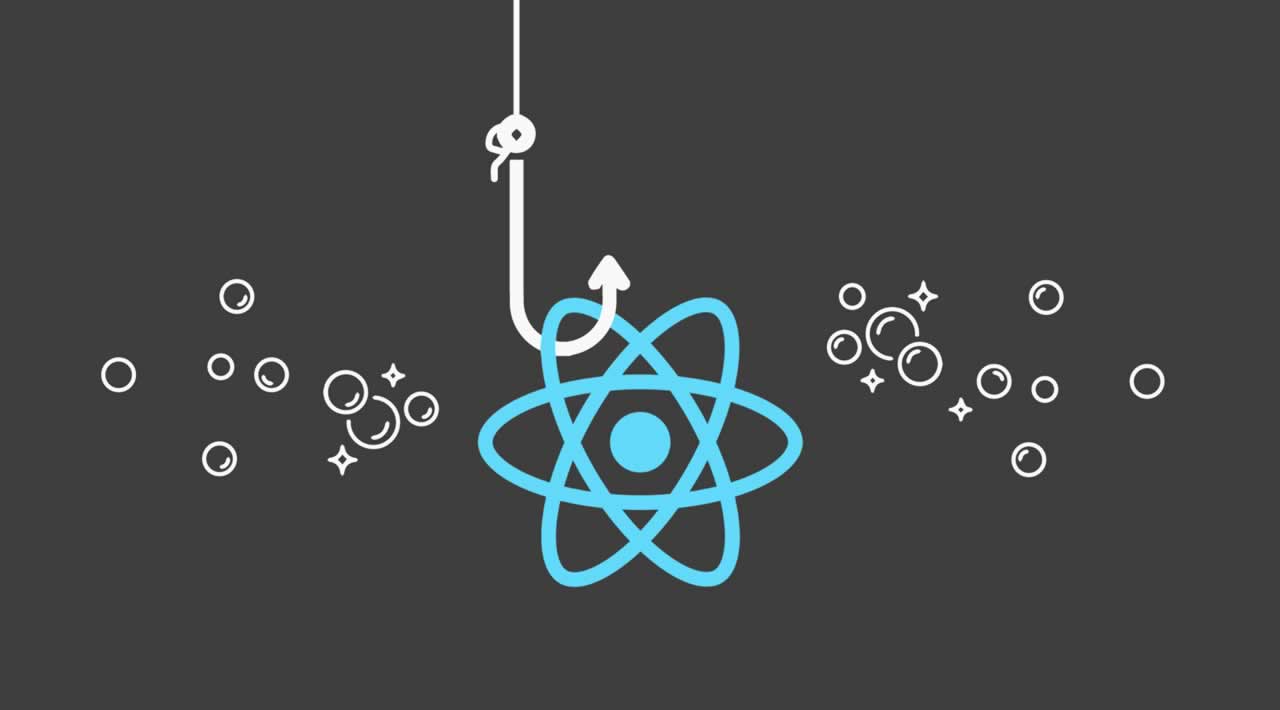
Have you ever found yourself writing the same code over and over again in different components? Do you wish there was a way to share stateful logic across your application without the need for props drilling or higher-order components? Look no further, because custom React hooks are here to save the day!
In this blog post, we'll be creating a custom hook called useFetch
that allows us to easily make HTTP requests and manage loading states within our components.
import { useState, useEffect } from 'react';
interface FetchState<T> {
data: T | null;
loading: boolean;
error: Error | null;
}
function useFetch<T>(url: string): FetchState<T> {
const [data, setData] = useState<T | null>(null);
const [loading, setLoading] = useState(false);
const [error, setError] = useState<Error | null>(null);
useEffect(() => {
async function fetchData() {
setLoading(true);
try {
const response = await fetch(url);
const json = await response.json();
setData(json);
} catch (e) {
setError(e);
} finally {
setLoading(false);
}
}
fetchData();
}, [url]);
return { data, loading, error };
}
With this custom hook, we can easily make HTTP requests and manage loading states within our components like so:
import { useFetch } from './useFetch';
function MyComponent() {
const { data, loading, error } = useFetch<User[]>('/users');
if (loading) return <p>Loading...</p>;
if (error) return <p>An error occurred: {error.message}</p>;
if (!data) return <p>No data</p>;
return (
<ul>
{data.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
Custom React hooks are a powerful tool that can help you share stateful logic across your components and improve the reusability and maintainability of your code. With just a few lines of code, you can create your hooks and use them to manage complex logic within your application.
In this blog post, we covered how to create a custom hook called useFetch
that allows us to make HTTP requests and manage loading states within our components. We saw how easy it is to use this hook and how it can help us avoid repeating the same code across our application.
Whether you're new to React or an experienced developer, custom hooks are a must-have in your toolkit. Give them a try and see how they can revolutionize the way you build your components!