Async componentDidMount
How to safely write async componentDidmount in your react application
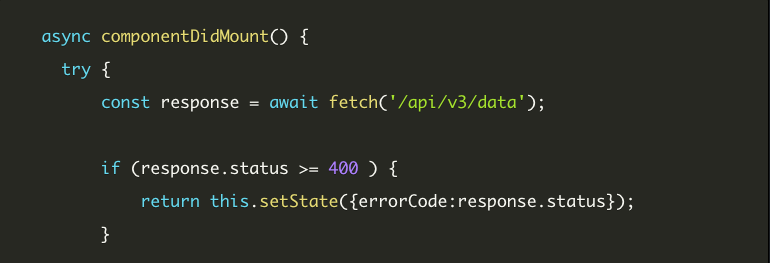
Async await is with us for a while now and it cleans our code from all the promise callbacks.
When we write code using async await we must understand that if a promise fails (Rejected) it will throw an exception and therefore we must safely write our code in a try catch block to be ready for when our promise is rejected.
Let's have a look async componentDidMount without try and catch block.
async componentDidMount() {
const response = await fetch('/api/v3/data');
const data = await response.json();
this.setState({data})
}
The code above could run smoothly in most cases and it will work but here is the catch.
What happens if server return status 400 ? we haven't verified the response status and what is going to happen, when we try to do response.json()
we get an exception and since we didn't use try and catch block, our app will crash.
It is very important to remember that fetch itself could result in a exception so you wont even get to the response.
Let's see how we can write this properly in the example below.
async componentDidMount() {
try {
const response = await fetch('/api/v3/data');
if (response.status >= 400 ) {
return this.setState({errorCode:response.status});
}
const data = await response.json();
return this.setState({data});
} catch(err) {
console.error(err) //only in development!
return this.setState({error:err});
}
}
We start by creating a try catch block inside our componentDidMount and then inside it we validate response.status in order to display proper UI in case we don't get a 200 or other green response. We can then safely continue to parse our JSON response without being scared of a crush since we catch any exception coming from our try block.
This is how you safely and simply write an async componentDidMount without the fear of an exception.